Rendering JSX on the Server with Fastify
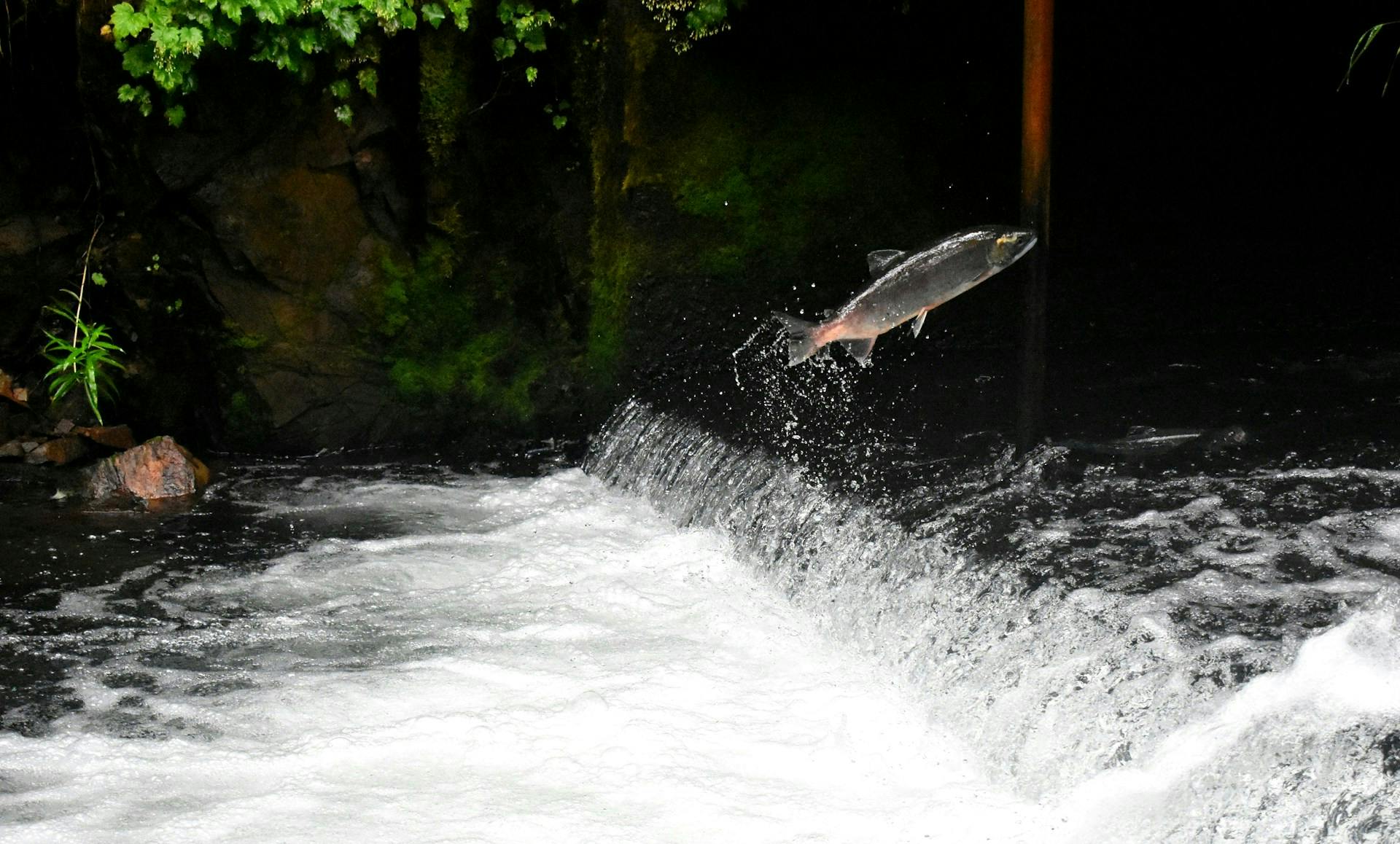
JSX is an excellent abstraction for building web interfaces. Introduced by Facebook and popularized by React, it's an extension of JavaScript designed to abstract nested function calls. It's expected that JSX code will be pre-processed (transpiled) into valid JavaScript before being executed in browsers or environments like Node.js.
Project Setup
First of all, let's start our project and install the necessary dependencies:
npm init -y
npm i fastify react react-dom
npm i -D @types/node @types/react @types/react-dom tsx typescript
Now, we set up the scripts for our project. The package.json
should look like this:
And this is the tsconfig.json
that we will use:
Creating our components
The React ecosystem already provides the necessary tools for rendering our components to HTML and sending them directly from the server to our client. So, first, let's create the root component:
And our home page:
Configuring Fastify to Render Our React Component
As we don't intend to load React to hydrate our HTML on the client side, we can use the renderToStaticMarkup
function exported from react-dom/server
. Our server initialization file will look like this:
If you start the project now (npm run dev
), you should see the page at http://localhost:3000. Of course, we can enhance our implementation by using the new streaming API, introduced in React 18 (which is the recommended method). To do that, we will make the following changes to our code:
And with that, we are able to render our React components on the server side and stream them to our client. Here is the link to the repository.