Using TypeScript in Node.js projects
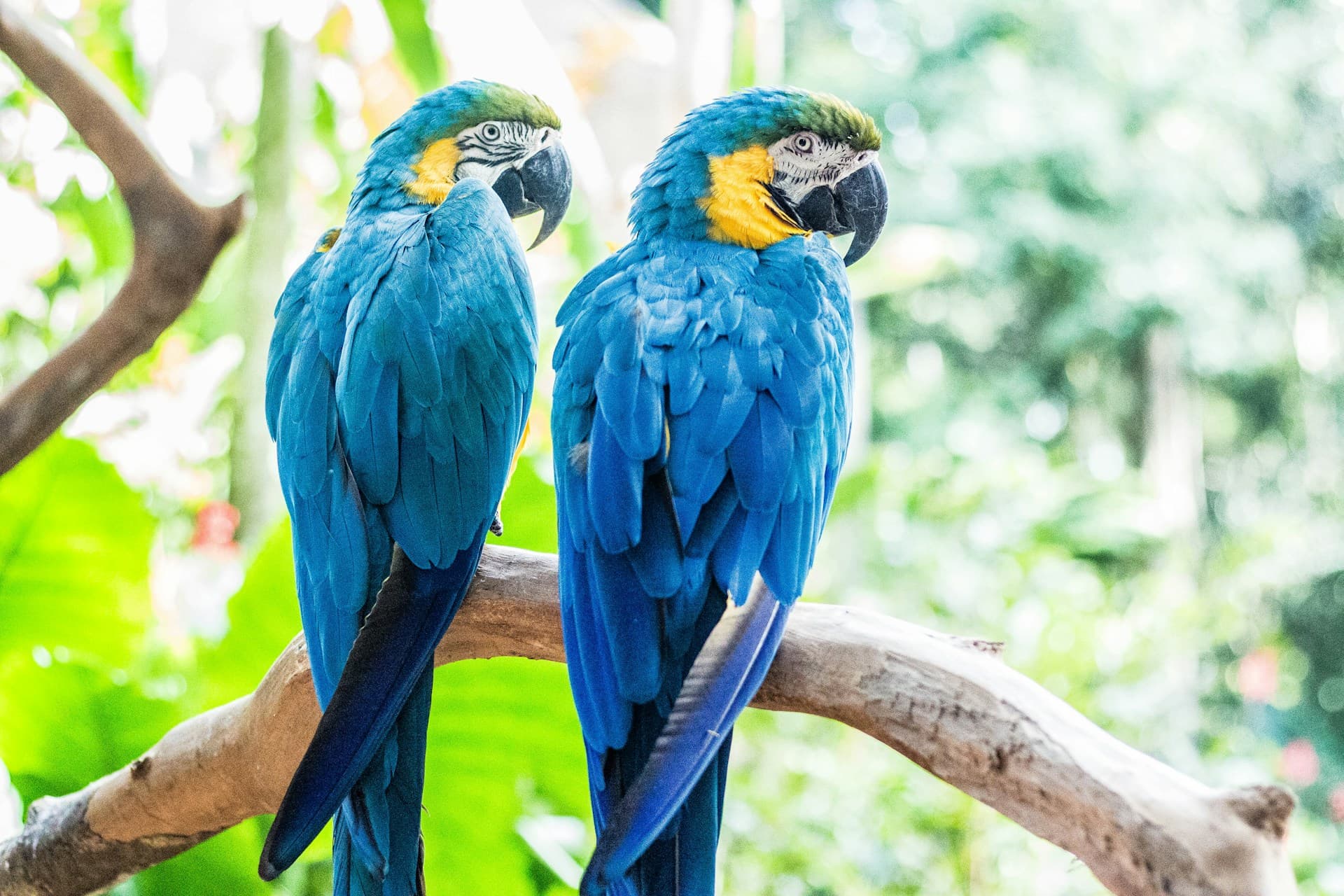
TypeScript is tremendously helpful while developing Node.js applications. Let's see how to configure it for a seamless development experience.
Setting up TypeScript
First, we need to install TypeScript. We can do this by running the following command:
Next, we need to create a tsconfig.json
file in the root of our project. This file will contain the TypeScript configuration for our project. Here is an example of a tsconfig.json
file that I picked from Total TypeScript and added a few more things (read the code and pay attention to the comments):
Setting up the build script
Next, we need to set up a build script that will compile our TypeScript code to JavaScript. First, install tsc-alias
to handle the aliases we defined in the tsconfig.json
file:
Then, you can add the build
script by adding the following script to our package.json
file:
Setting up the development script
Next, we need to set up a development script that will watch for changes in our TypeScript files and recompile them. Personally, I like to use tsx
, as it provides a much faster development experience compared to the built-in TypeScript watcher or ts-node. First, install tsx
:
Then, you can add the dev
script (in order to start the project in development mode) by adding the following script to your package.json
file:
Yes, you won't get typechecks while developing using tsx
, but you can run npm run build
for that or add a new typecheck
scripts to your package.json
, and run it whenever you want to check for type errors: